Main Content
Graphics Programming (Winter term 2024/2025)
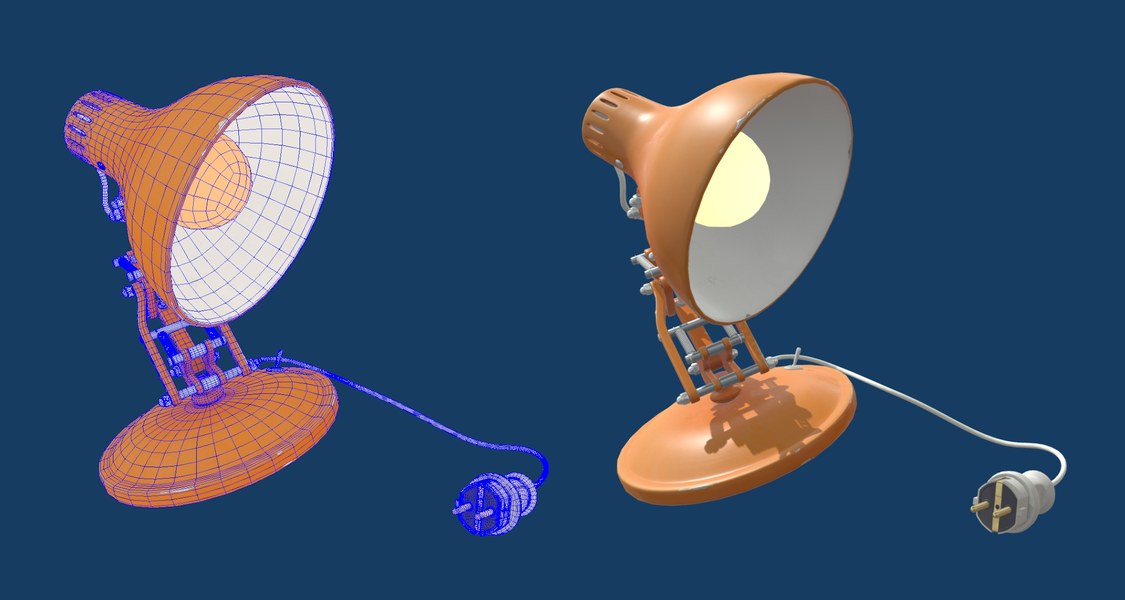
Lecturer: Prof. Dr. Thorsten Thormählen
Module name: CS 581
Language of lecture: German
Qualification objectives
The lecture Graphics Programming teaches how to create interactive computer graphics applications. This includes the general concepts of computer graphics, which are independent of the programming environment, such as the mathematical description of 2D and 3D objects, lights and cameras, the representation of the 3D scene in a hierarchical scene graph, or a mathematical description of materials and their reflectance properties. By programming examples in a practice-oriented programming environment, the participants learn all the techniques necessary to independently implement their own computer graphics project in the future. To this end, also basics for creating graphical user interfaces are taught. In addition, the course aims at improving the overall ability of the participants to perform scientific work, to solve problems, and to communicate.
Programming environment
The course puts a strong focus on the modern rasterization pipeline and uses OpenGL as a graphic library and GLSL as the shader language. Basic knowledge of object-oriented programming is required. Programming exercises and the examples shown in the lecture are presented in Java. Alternatively, all implementations are also given in C++ and several interactive demonstrations in WebGL/JavaScript are provided.
Organisation
The course consists of a lecture (4 hours per week) and exercises (2 hours per week).
Lecture: Mon 08h30 - 10h00, Fri 10h15 - 11h45, (H | 04) Hörsaal III A3
Exercise: Mondays after the lecture, (H | 04) Hörsaal III A3
Tutors: Felix Nitzsche, Mohamed Eldakar
Course structure
- Introduction
- Graphical user interfaces
- Representation and rendering of 2D objects
- OpenGL pipeline
- Object transformations
- Cameras
- Textures
- Buffer Objects
- OpenGL Shading Language (GLSL)
- Light and materials
- Shadows
- Game Programming
Lecture slides
The lecture slides are available as HTML5 websites. It is recommended to use a modern browser (such as Google Chrome, Mozilla Firefox). In Google Chrome, the browser's print function can be used to create a PDF file of the slides.
Currently, not all slides are translated to English (see below). All slides and more details can be found at the German version of this page.
There are two versions of the slides: internal and external. The internal version comprises additional slides that are only available to the students of the University of Marburg. The login will be announced in the lecture.
Part | Chapter | Link |
---|---|---|
1. Introduction | 1.1 Introduction | external, internal |
1.2 Organization | external, internal | |
2. GUIs | 2.1 Graphical User Interfaces | external, internal |
2.2 GUIs with Java | external, internal | |
2.3 GUIs with Java (Android) | external, internal | |
2.4 GUIs with C++ and Qt | external, internal | |
3. 2D Objects | 3.1 Vector and Raster Graphics | external, internal |
3.2 Raster Conversion | external, internal | |
4. OpenGL Pipeline | 4.1 OpenGL Pipeline | external, internal |
5. Object Transformations | 5.1 2D Transformations | external, internal |
5.2 3D Transformations | external, internal | |
6. Cameras | 6.1 Perspective Projection | external, internal |
6.2 Parallel Projection | external, internal | |
7. Textures | 7.1 Textures | external, internal |
8. Buffer Objects | 8.1 Buffer Objects | external, internal |
9. OpenGL Shading Language | 9.1 OpenGL Shading Language (GLSL) | external, internal |
10. Light and Materials | 10.1 Light and Materials | external, internal |
10.2 Image-based Lighting (IBL) | external, internal | |
11. Shadows | 11.1 Shadows | external, internal |
11.2 Ambient Occlusion | ||
12. Game Programming | 12.1 Graphics Engine | |
12.2 Shader Effects |
Exercise Class
Registration for the exercise class and submission of the assignments is done via the ILIAS learning platform.
Video Tutorials on Shader Programming
In the winter term 2021 we started to publish videos on shader programming. Especially the first videos of this series have a strong link to the material of this lecture.
- What are Shaders? A Hands-on Introduction [Shaders Monthly #1, 11/2021]
- Perspective Projection in GLSL [Shaders Monthly #2, 12/2021]
- OpenGL Modelview Matrix and 3D Transformations [Shaders Monthly #3, 01/2022]
- Blinn Phong Shading: Theory and Implementation [Shaders Monthly #4, 02/2022]
- Texture Mapping in GLSL [Shaders Monthly #5, 03/2022]
- What are Mipmaps? Texture Filtering in GLSL [Shaders Monthly #6, 04/2022]
- Procedural Textures: A Practical Introduction [Shaders Monthly #7, 05/2022]
- Procedural Noise: Value and Gradient Noise in GLSL [Shaders Monthly #8, 06/2022]
- Microfacet BRDF: Theory and Implementation of Basic PBR Materials [Shaders Monthly #9, 07/2022]
- Importance Sampling: Image-based Lighting of a Lambertian Diffuse BRDF [Shaders Monthly #10, 09/2022]
- Image-based Lighting (IBL) of PBR Materials [Shaders Monthly #11, 01/2023]
- Halton Low-Discrepancy Sequence [Shaders Monthly #12, 5/2023]
- Sampling of Environment Maps for Image-based Lighting [Shaders Monthly #13, 9/2023]
- Deferred Shading [Shaders Monthly #14, 3/2024]
Code Examples
The lecture slides contain several code examples. These are listed here:
3.1 House2D (Java)
3.1 MyBufferedImage (Java)
4.1 FirstTriangle (Java, Qt, Glut)
5.1 TriangleTransform (Java, Qt, Glut)
5.2 GimbalLock (Java, Qt, Glut)
5.2 RotationInterpolation (Java, Qt, Glut)
6.1 Dolly Zoom (Java, Qt, Glut)
6.1 LookAt (Java, Qt, Glut)
6.1 LookAtLocalTrans (Java, Qt, Glut)
6.1 Viewport (Java, Qt, Glut)
6.1 ZFighting (Java, Qt, Glut)
6.2 Axonometric (Java, Qt, Glut)
6.2 Oblique (Java, Qt, Glut)
7.1 Texture (Java, Qt, Glut)
7.1 TextureParameter (Java, Qt, Glut)
7.1 TextureBlend (Java, Qt)
7.1 Texture3D (Java, Qt, Glut)
7.1 Fbo (Java, Qt, Glut)
7.1 FboDepth (Java, Qt, Glut)
8.1 VboDrawArrays (Java, Android, Qt, Glut)
8.1 VboDrawElements (Java, Android, Qt, Glut)
8.1 VboUpdate (Java, Android, Qt, Glut)
8.1 VboStride (Java, Android, Qt, Glut)
8.1 Vao (Java, Qt, Glut)
8.1 ObjToVboExample (Java, Android, Qt, Glut)
8.1 FirstForwardCompatible (Java, Qt, Glut)
9.1 FirstShader (Java, Android, Qt, Glut, WebGL, GSN Composer)
9.1 ShaderUniform (Java, Android, Qt, Glut, WebGL, GSN Composer)
9.1 ShaderNormalTrans (Java, Android, Qt, Glut, WebGL, GSN Composer)
9.1 ShaderTexture (Java, Android, Qt, Glut, WebGL, GSN Composer)
10.1 ShaderLightMat (Java, Android, Qt, Glut, WebGL, GSN Composer)
10.1 StoneDemonPhong (GSN Composer)
10.1 ShaderMicrofacetBrdf (Java, Qt, Glut, WebGL, GSN Composer)
10.1 MicrofacetSpheres (GSN Composer)
10.1 ShaderPhongPointLight (GSN Composer)
10.1 ShaderPhongSpot (Java, Qt, Glut)
10.2 EnvmapLighting (GSN Composer)
10.2 SpheresIBLRef (GSN Composer)
10.2 SpheresIBL (GSN Composer)
10.2 ImageBasedLighting (GSN Composer)
11.1 PlanarShadow (GSN Composer)
11.1 PlanarShadowAmbient (GSN Composer)
11.1 ShadowMap (GSN Composer)
Note: This list is currently incomplete and will be updated.
For some code examples the tool ObjToVbo.cpp is required, which converts a 3D model in Wavefront OBJ format into an array of floats and saves it into a text or binary file. Such a float array can be read with high speed and with little programming effort and can be passed directly to a VBO in OpenGL.
Interactive demonstrations
3.2 Polygon Rasterization (demo)
10.1 Phong / Blinn Phong Shading (demo)
10.2 Importance Sampling of a Hemisphere (demo)
12.1 Ear Cutting (demo)